How to Delete Multiple Entries That Match a Given Regex in FaunaDB

Jose Nicholas Francisco
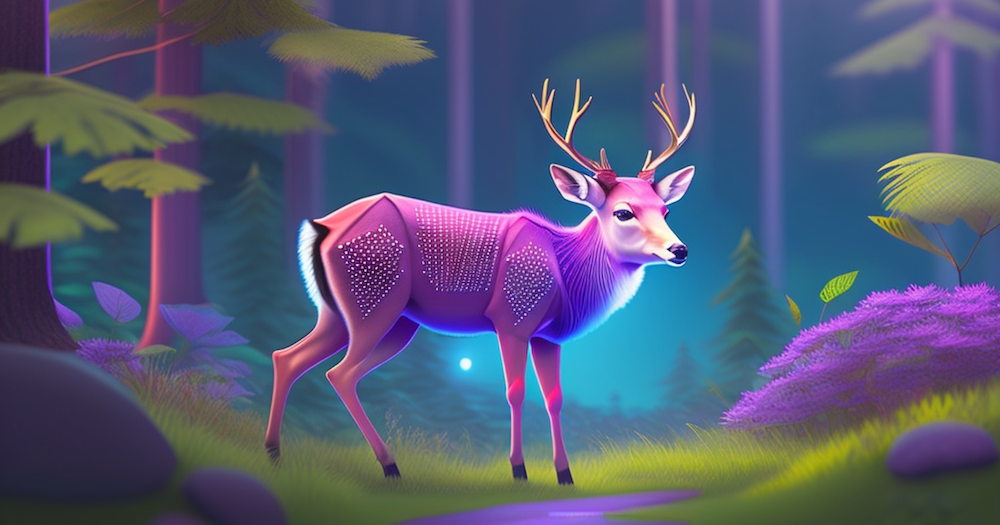
This blog post should be a quick one.
Long story short, I have a database in Fauna that contains loads upon loads of URLs. And for a reason I shall not disclose—though you are welcome to playfully speculate—I had to delete quite a few URLs from this database.
Here’s the problem: Fauna’s documentation sucks has room for improvement.
So allow me to spare you the half-hour it took me to figure out how to delete multiple entries from a database in Fauna.
Really all you have to do is
Grab your `secret`, the name of your table, and your regex and plop them into the script below.
Profit.
I highly recommend printing the results first before deleting anything just to be sure that `results` contains the entries you indeed wish to delete. But beyond that, there’s not much else to do. The script below honestly boils down to initializing fauna and running a for-loop that deletes the entries that match the regex.
const faunadb = require('faunadb')
const q = faunadb.query
const regex = /YOUR REGEX HERE/
const table = “table name here”
const runner = async () => {
const client = new faunadb.Client({
secret: 'YOUR_SECRET_HERE',
})
const results = await client.query([
q.Map(
q.Paginate(q.Documents(q.Collection(table)), { size: 10000 }),
q.Lambda(table, q.Get(q.Var(table))),
),
])
results[0].data.forEach(async element => {
if(element.data.target.match() {
await client.query([
q.Delete(element.ref)
])
}
});
}
runner();
If you use fauna, I hope you found this helpful! I know I sure did. I’m currently keeping this script in my back pocket for a rainy day.