A Guide to Respectful API Back-Off Strategies
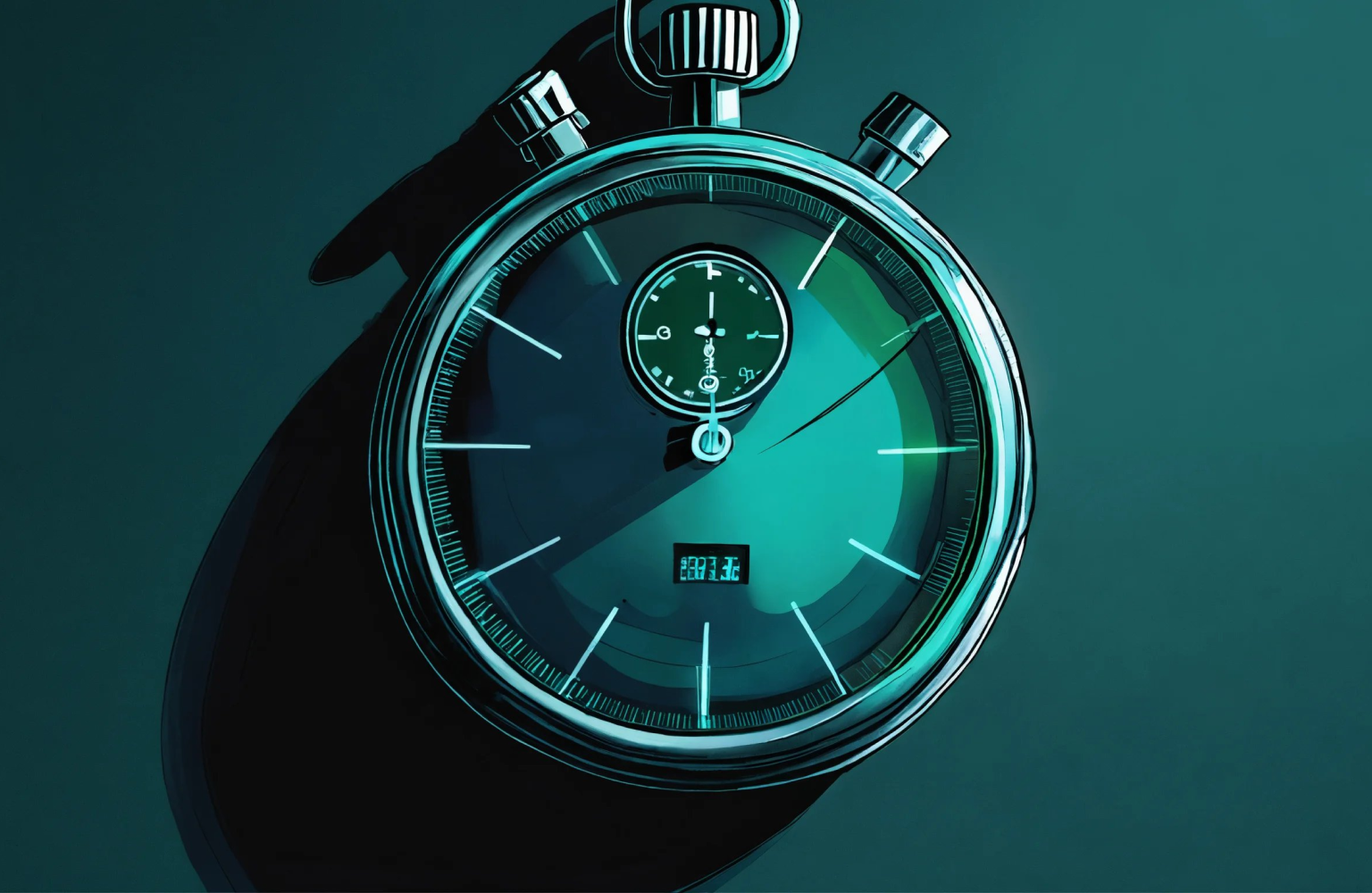
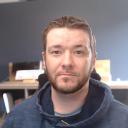
When developing apps, using AI services like Deepgram can take them to the next level. Deepgram helps you understand speech with advanced tech. But, there's a catch - you've got to play nice with how often you ask Deepgram for help, or you might get a timeout. This guide will show you how to avoid those timeouts by slowing down your requests just right, keeping everything running smoothly for you and your users. Let's dive in on how to be a good digital neighbor by handling those "slow down" messages, known as 429 errors, with grace.
Understanding 429 Response Errors
Before diving into back-off strategies, it's essential to understand what a 429 response error is. Think of a 429 error like someone saying, "Hold up, you're asking me too many questions too fast!" Deepgram sends this when your app sends too many requests in a short time. It's Deepgram's way of making sure everyone gets a turn without overwhelming the system.
Why Implement Back-Off Strategies?
Implementing a back-off strategy is crucial for several reasons. First, it helps avoid hitting the rate limit, which can disrupt your service and affect user experience. Second, it demonstrates good citizenship in the API ecosystem by ensuring that your application does not monopolize shared resources. Finally, it can prevent your API key from being temporarily blocked, which could lead to significant downtime.
Step-by-Step Guide to Implementing Back-Off Strategies
I'll attempt to guide us through Node.js and Python examples.
Step 1: Making a Request to Deepgram
In Node.js, you'd make a request using node-fetch like so.
Python, would be similar using requests.
Step 2: Detecting 429 Errors
The first step in implementing a back-off strategy is to detect 429 errors. This can be done by monitoring the HTTP status codes of responses from the Deepgram API. If a response returns a 429 status code, it's an indication that your request rate is too high.
In JavaScript;
In Python;
Step 3: Implementing a Basic Back-Off Strategy
A basic back-off strategy involves waiting for a predetermined amount of time before retrying the request. For example, if you receive a 429 error, you might wait for one minute before sending another request. It's essential to ensure that this delay increases exponentially with each subsequent 429 response, to reduce the chance of hitting the rate limit again. This is known as an "exponential back-off" strategy.
In JavaScript;
In Python;
Step 4: Advanced Strategies: Jitter and Rate Limit Optimization
For more sophisticated back-off strategies, consider implementing jitter—a random variation in the wait time—and monitoring your request rate to adjust it dynamically based on the rate limit information provided by Deepgram. Jitter helps to spread out retries from different instances of your application, reducing the likelihood of overwhelming the API after the wait period.
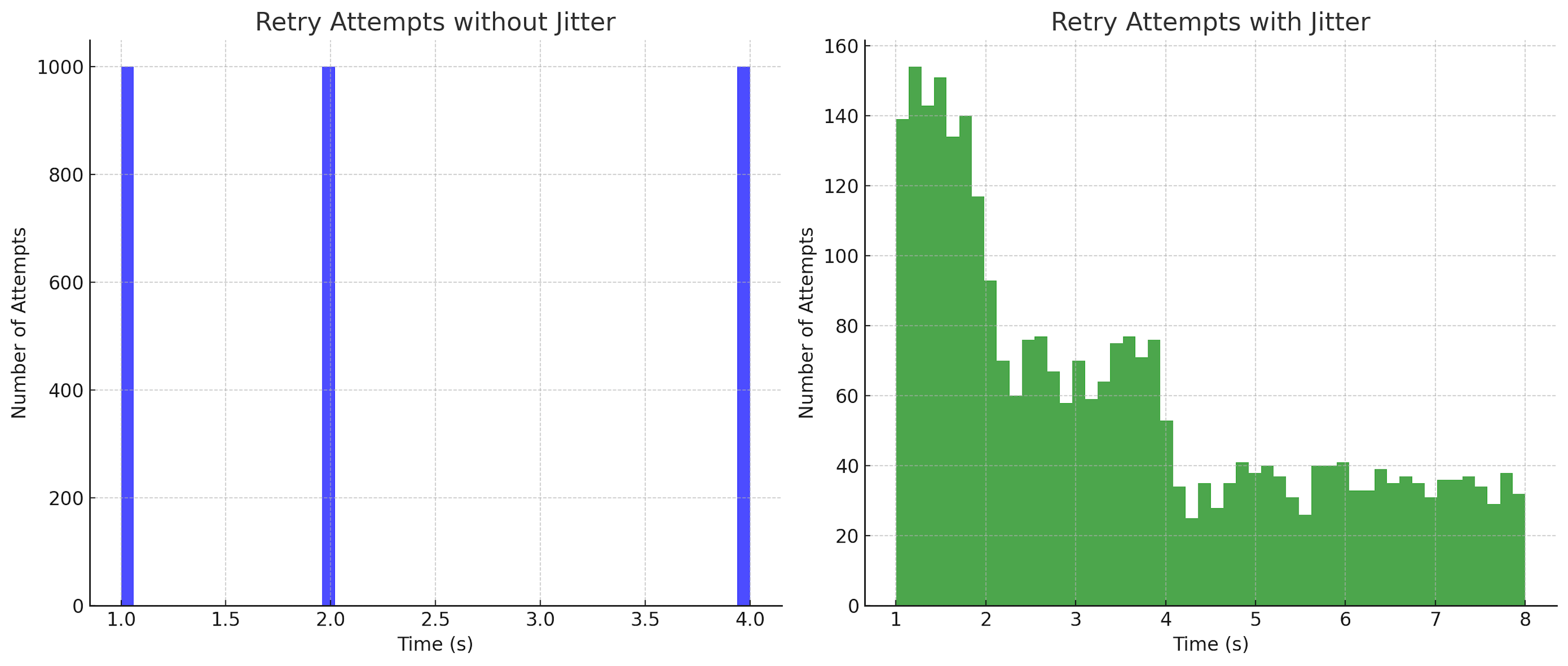
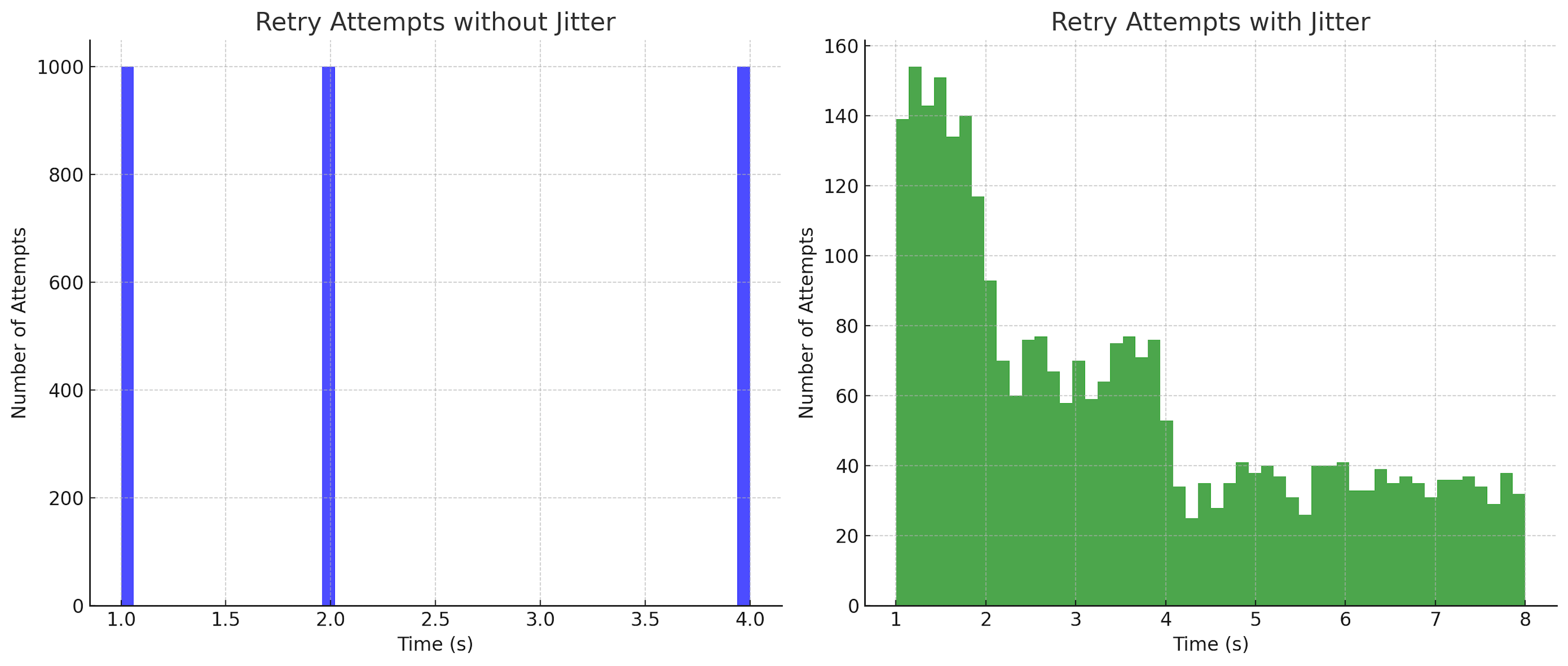
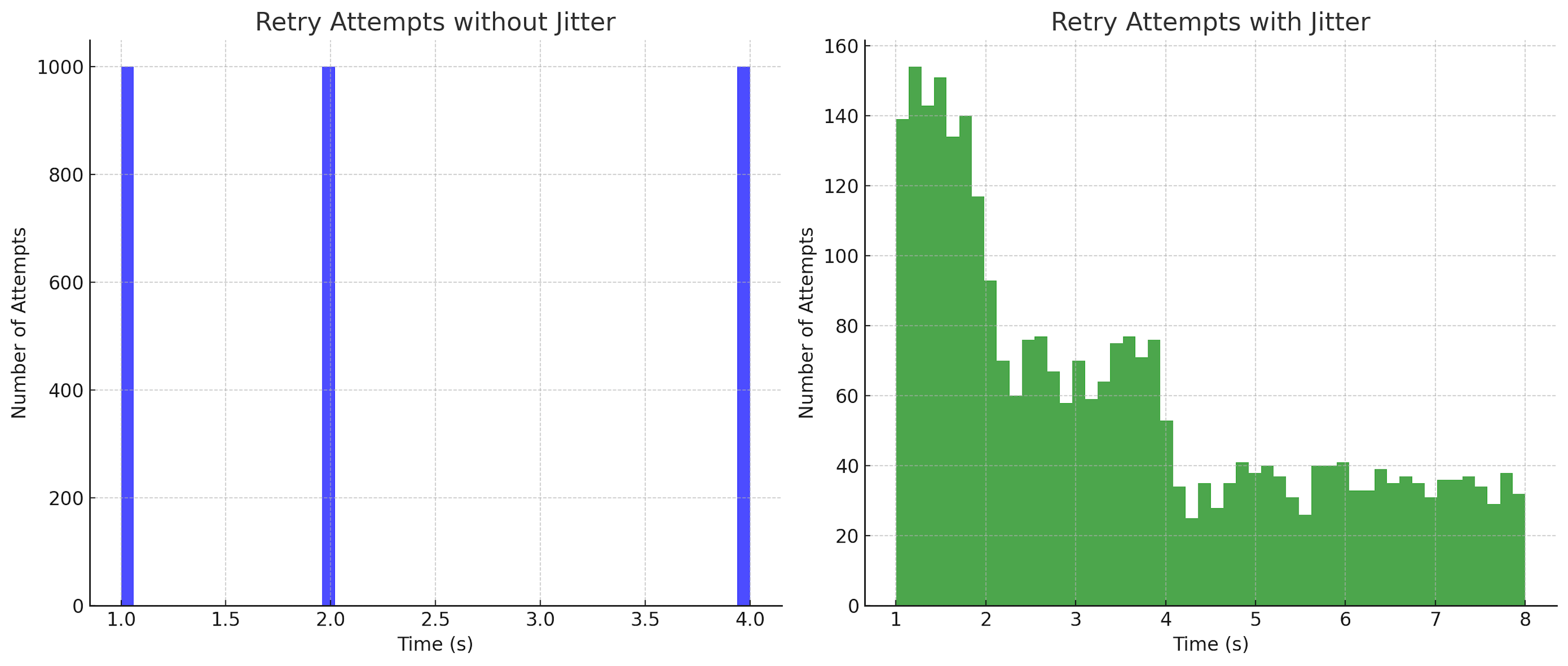
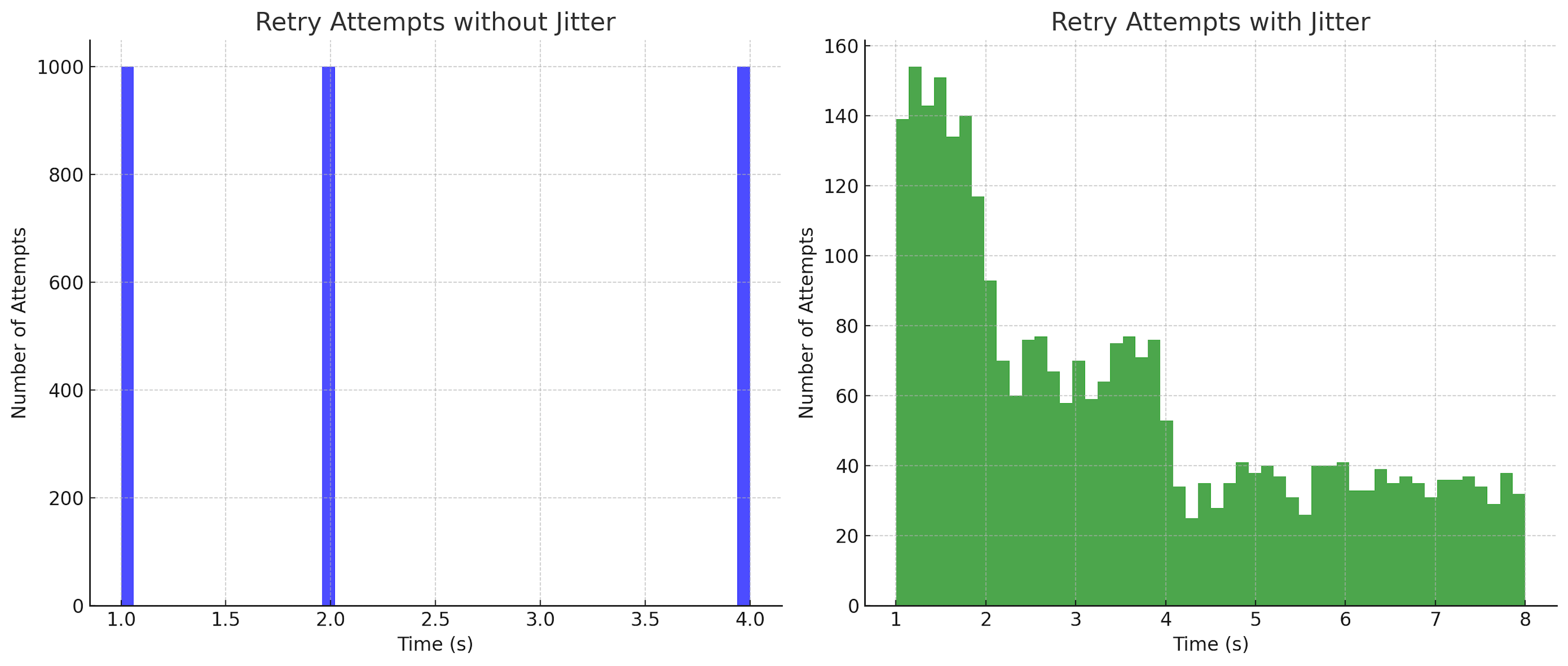
In JavaScript;
In Python;
Step 5: Logging and Monitoring
Implement logging and monitoring of your API usage and the occurrences of 429 errors. This data can be invaluable for adjusting your request patterns and back-off logic to stay within rate limits while meeting your application's needs.
Conclusion
Respecting API rate limits is a critical aspect of integrating with services like Deepgram. By implementing thoughtful back-off strategies, you can ensure that your application remains efficient, reliable, and respectful of shared resources. Remember, the goal is to provide a seamless experience for your users while coexisting harmoniously with other applications in the API ecosystem. Happy coding!
Sign up for Deepgram
Sign up for a Deepgram account and get $200 in Free Credit (up to 45,000 minutes), absolutely free. No credit card needed!
Learn more about Deepgram
We encourage you to explore Deepgram by checking out the following resources:
Unlock language AI at scale with an API call.
Get conversational intelligence with transcription and understanding on the world's best speech AI platform.