Auto-Playback Across Devices (especially iOS)
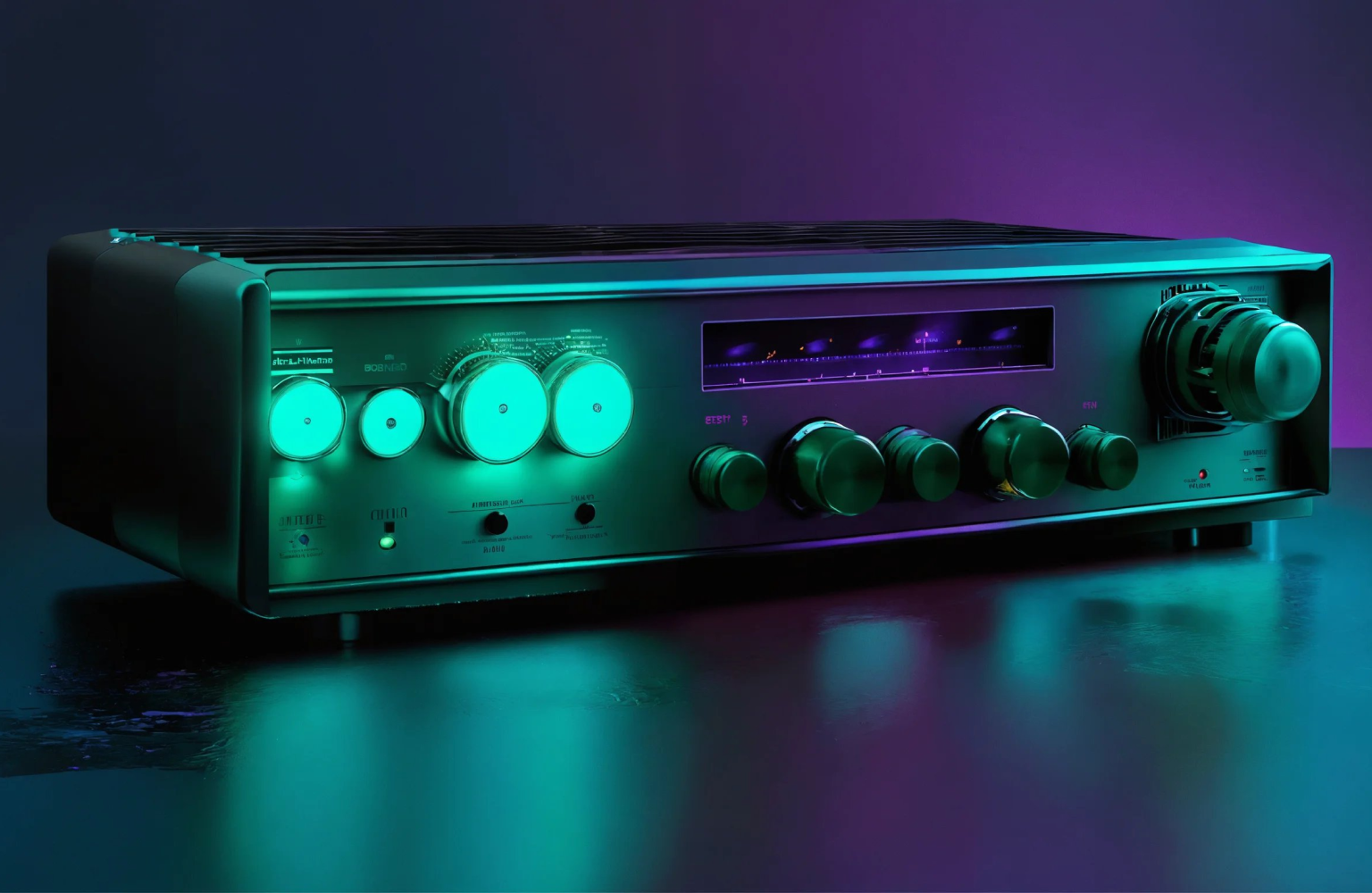
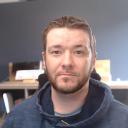
In the world of web development, creating an immersive user experience often involves the seamless integration of audio content. Whether it's background music, podcast episodes, or notification sounds, audio plays a pivotal role in engaging users. However, developers frequently face hurdles when implementing auto-play audio features, especially when ensuring compatibility across various browsers and devices. This post aims to demystify the process, guiding you through the steps to auto-play audio in any browser on any device using the `<audio>` element, with a special nod to vanilla JavaScript. Additionally, we'll introduce a handy React package for those looking to streamline their development process.
Embracing Cross-Browser/Cross-Device Compatibility
One of the main challenges in auto-playing audio content lies in the varying support across browsers and devices, particularly on iOS devices, where autoplay restrictions are stricter. Historically, the Web Audio API has been a go-to for developers seeking rich audio experiences on the web. However, it's not without its cross-browser quirks, especially concerning auto-playback.
Switching our focus to the <audio> element simplifies matters significantly. Unlike the Web Audio API, the <audio> element ensures a greater degree of compatibility across different environments. According to Mozilla Developer Network, leveraging the <audio> element over the Web Audio API can resolve numerous cross-browser issues, including the challenge of auto-playback on iOS browsers. This makes the <audio> element a reliable choice for developers aiming for broad compatibility without the need for extensive browser-specific adjustments.
## Implementing Audio Sprites
An effective strategy to optimize audio playback and ensure compatibility is the use of audio sprites. This technique involves combining multiple audio clips into a single file and playing specific sections as needed. It's akin to image sprites used in graphics rendering but for audio. Audio sprites can significantly reduce the number of server requests and ensure smoother playback.
For instance, we can utilize a single, tiny silent MP3 file as a placeholder for audio we receive from API requests. This method, highlighted by Remy Sharp, involves using a base64-encoded audio sprite, such as the following example:
This sprite serves as a universal placeholder, mitigating issues related to audio initiation across different platforms.
Best Practices for Audio Playback
When implementing auto-play audio, it's crucial to adhere to best practices to ensure a smooth user experience and avoid common pitfalls. The Mozilla Developer Network offers valuable insights:
User Engagement: Always initiate audio playback in response to a user action, such as a click or tap. This approach aligns with the autoplay policies of most browsers and enhances user experience by preventing unexpected audio from playing.
Optimize Resources: Utilize audio sprites to minimize the number of server requests and ensure seamless playback.
Volume Control: Provide users with the ability to control audio volume, ensuring that your website or application remains user-friendly.
Compatibility Checks: Regularly test your audio implementation across different browsers and devices to identify and address any compatibility issues.
To create a web application or site that gracefully handles audio across all browsers and devices, understanding and implementing best practices is crucial. Let's delve deeper into each of the previously mentioned strategies, providing actionable insights for developers aiming to enhance their audio features.
1. User Engagement
Autoplay policies have evolved to prioritize user experience, often requiring a user interaction to start audio playback. This development reflects a broader shift towards user-centric design, where user consent and engagement are paramount. To align with these practices:
Interactive Audio Initiation: Design your UI to encourage users to initiate audio playback actively. For instance, a play button that's prominently displayed can serve as a clear invitation for users to engage with your audio content.
Contextual Autoplay: If autoplay is essential for your application, consider triggering it only after a user has interacted with your site in some way, such as clicking a link or completing a form. This approach ensures that when the audio starts, it feels like a natural part of the user's journey.
2. Optimize Resources
Efficient resource management is key to a smooth, fast-loading web experience. Audio sprites play a significant role here, but there's more to resource optimization:
Lazy Loading: Implement lazy loading for your audio files, ensuring they are loaded only when needed. This reduces initial load times and conserves bandwidth.
Compression: Use compressed audio formats (like AAC or MP3) and optimize your audio sprites to balance quality and file size, minimizing download times without sacrificing audio quality.
3. Volume Control
Providing users with control over audio volume is not just a courtesy; it's a necessity for user-friendly design. Implementing accessible volume controls allows users to tailor the audio experience to their environment and preferences:
Accessible Controls: Ensure volume sliders or controls are easily accessible and clearly labeled, helping users adjust the volume without frustration.
Remember User Preferences: Utilize local storage to remember users' volume settings across sessions, making your site more accommodating and personalized.
4. Compatibility Checks
Cross-browser and cross-device compatibility is a cornerstone of web development. Regular testing and adaptation are required to ensure that everyone, regardless of how they access your site, enjoys a seamless audio experience:
Use Feature Detection: Implement feature detection to gracefully handle differences in audio support across browsers. This can help you provide alternative content or instructions for browsers that might not support certain audio features.
Responsive Design: Ensure your audio controls and elements are responsive, accommodating various screen sizes and orientations. This is particularly important for mobile devices, where screen real estate is limited.
Continuous Testing: Regularly test your audio implementation on multiple devices and browsers, including mobile platforms and older browser versions. Tools like BrowserStack can simulate a wide range of environments, helping you identify and fix compatibility issues.
Incorporating these best practices into your web development process can significantly enhance the user experience of your audio-enabled web applications or sites. By focusing on user engagement, resource optimization, volume control, and compatibility checks, you'll be well on your way to creating more immersive and user-friendly audio experiences.
Leveraging React for Audio Playback
When building modern applications with React, incorporating audio features can often involve navigating a complex landscape of browser compatibilities, user engagement strategies, and performance optimizations. Our react-nowplaying NPM package streamlines this process, encapsulating the best practices and technical strategies outlined earlier into a simple, easy-to-use package. This means developers can focus more on creating immersive audio experiences and less on the underlying complexities.
The react-nowplaying package abstracts away the intricacies of audio playback, handling user engagement requirements, resource optimizations, volume control mechanisms, and ensuring cross-browser and cross-device compatibility. By integrating this package into your React application, you're leveraging a solution that's been crafted with these best practices in mind, allowing you to provide a seamless audio experience with minimal effort.
Getting Started
Installation
Add to Your Project
First, import the React context provider into your application:
Wrap the necessary part of your application with the NowPlayingContextProvider to make it aware of the audio context:
Play Audio Content
The react-nowplaying package simplifies playing audio, whether from a Blob or a URL:
Play Blob Data
Play a URL String
Audio Controls and Events
With react-nowplaying, you gain access to the native audio functionality, plus some to intuitive controls like pause and resume.
By integrating react-nowplaying into your React projects, you benefit from a comprehensive solution that addresses the complexities of audio playback in web applications, allowing you to focus on delivering content and creating engaging user experiences.
You can read more about our React NowPlaying package on NPM.
Sign up for Deepgram
Sign up for a Deepgram account and get $200 in Free Credit (up to 45,000 minutes), absolutely free. No credit card needed!
Learn more about Deepgram
We encourage you to explore Deepgram by checking out the following resources:
Unlock language AI at scale with an API call.
Get conversational intelligence with transcription and understanding on the world's best speech AI platform.