Log Deepgram Call Summaries In Salesforce
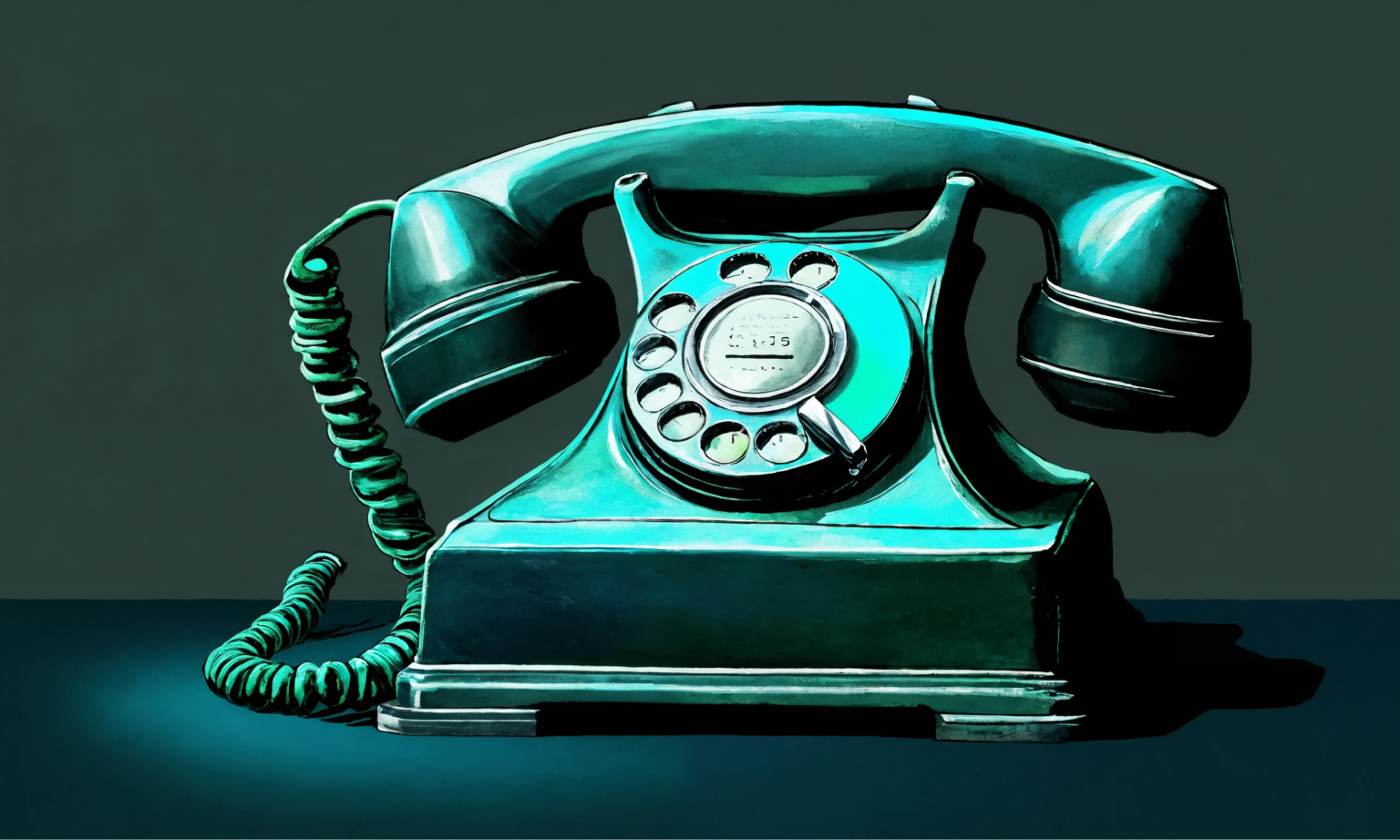
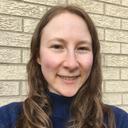
Introduction
Many companies use Salesforce as a source of truth for a wide variety of business activities and processes. You may be wondering how you can incorporate Deepgram to add richer information into Salesforce.
There are infinite variations on how companies customize their Salesforce instance; this blog post will demonstrate one example use case: summarizing sales calls using Deepgram, and logging the call summaries on a Salesforce opportunity and account.
Check out the GitHub repo that goes along with this post here.
A crash course in Salesforce terminology
Salesforce has its own world of concepts and relationships, and if you’re already in that world, then you’ll likely be familiar with all of the terms in this example. For readers newer to Salesforce, and to ensure a common understanding, here are some key concepts used throughout this example.
Account: A profile record of a single customer, which could be either a company or an individual.
Opportunity: A deal in progress with a specific account.
Activity: A task, event, or calendar that happens in relation to progressing forward on an opportunity for an account. Employees perform activities in order to close a deal; this can involve calls, meetings, research, form submissions, page views, document signings, and more. In this example, we’ll be focused on call activities.
Apex: Salesforce’s own programming language, offering an interface for CRUD operations (create, read, update, delete) on Salesforce objects, plus richer features that won’t be needed here.
Set up your Salesforce API credentials
Salesforce requires three pieces of information to use its API: your email, password, and an activity token.
Log into Salesforce as usual, noting the email and password you use to log in. Then follow their instructions to reset your security token, which will send you an email with your security token.
Now using your email, password, and security token, you will be able to use the Salesforce API. Those credentials will be filled in as static variables in the starter code.
Use the Deepgram API to transcribe a call with summarization
Ensure you have the Deepgram Python SDK installed via the command line:
This example provides a sample audio file, or you can provide a different URL to your own audio.
You’ll also need to provide your own Deepgram API key.
Finally, specify the name of an existing Salesforce account. This example assumes you have an account named “Deepgram.” You can create a new account for testing, or use an existing one.
With those pieces in place, you’ll summarize the call audio via a Deepgram API call with our summarization feature:
Retrieve the text summary from the call
The Deepgram API response JSON will contain the summary at the following path:
Identify the Salesforce Account and Opportunity records to update
The simple-salesforce Python package is an open-source library which enables you to work with the Salesforce Apex API to run queries to create, read, update, and delete records. This example involves reading and updating account and opportunity records, and creating call activity records.
First, install the package on the command line:
Then in Python, import the package, and create an authenticated client:
Query for the account ID given the name of the account the call was for:
Then use the account ID to get the latest opportunity for the account. If you prefer, you can modify the logic to select a different opportunity besides the latest one.
Write the Deepgram call summary to the Salesforce record
Now that you have the Salesforce IDs of the account and opportunity, you’re ready to log a new call event to the account and opportunity with the Deepgram call summary and today's date. You can customize this even further by setting your own date and call duration.
This example makes the basic assumption that all calls are 60 minutes long, and just ended now. You can also customize the call’s subject to include any other information you prefer.
Viewing call logs with summaries from the Salesforce UI
Within the opportunity, in the Activity tab, you’ll see the call activity. When you toggle to display the activity’s complete information, you’ll see the Deepgram call summary in the description (shown in the screenshot below). You’ll similarly see the call in the activity log on the account.
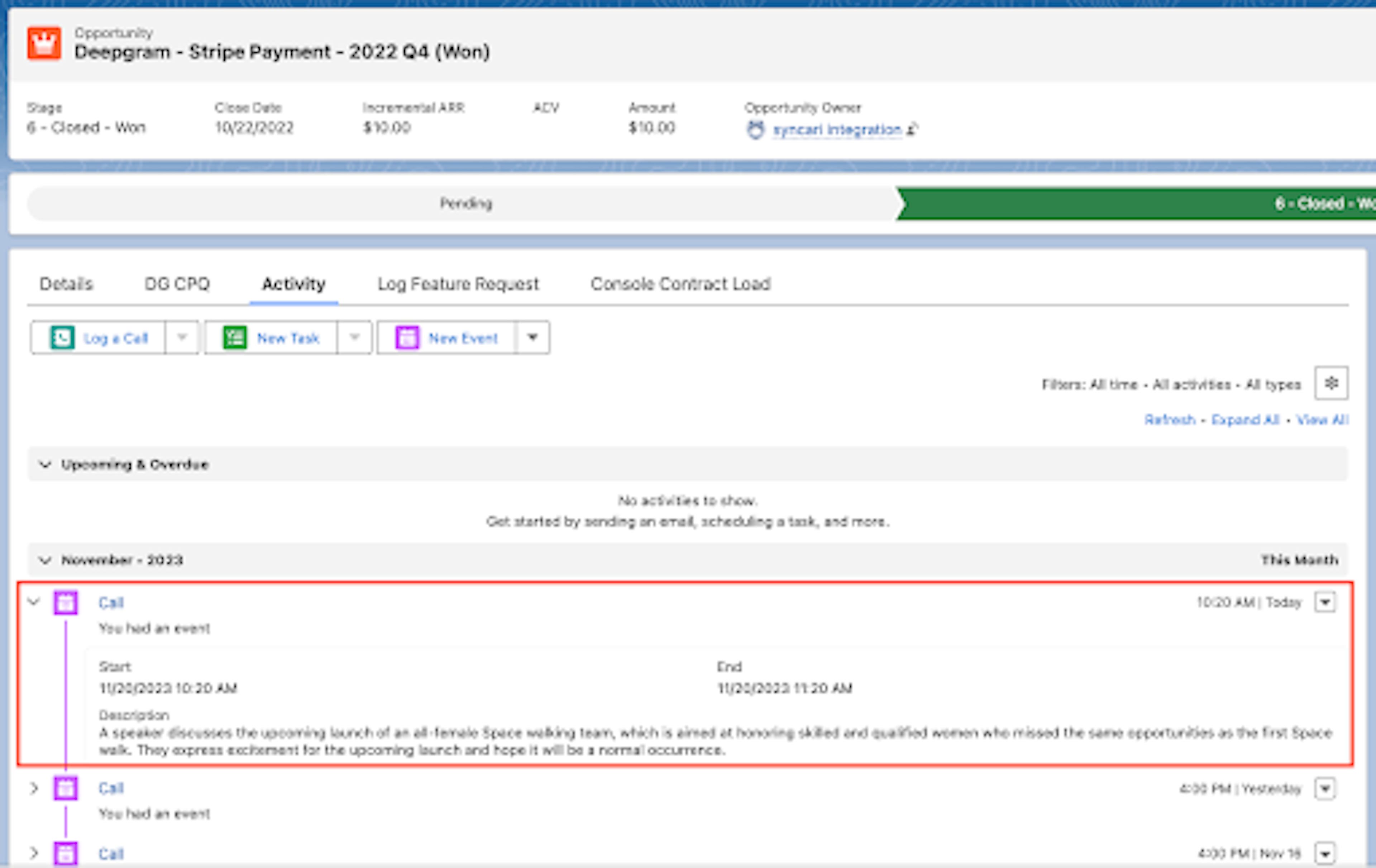
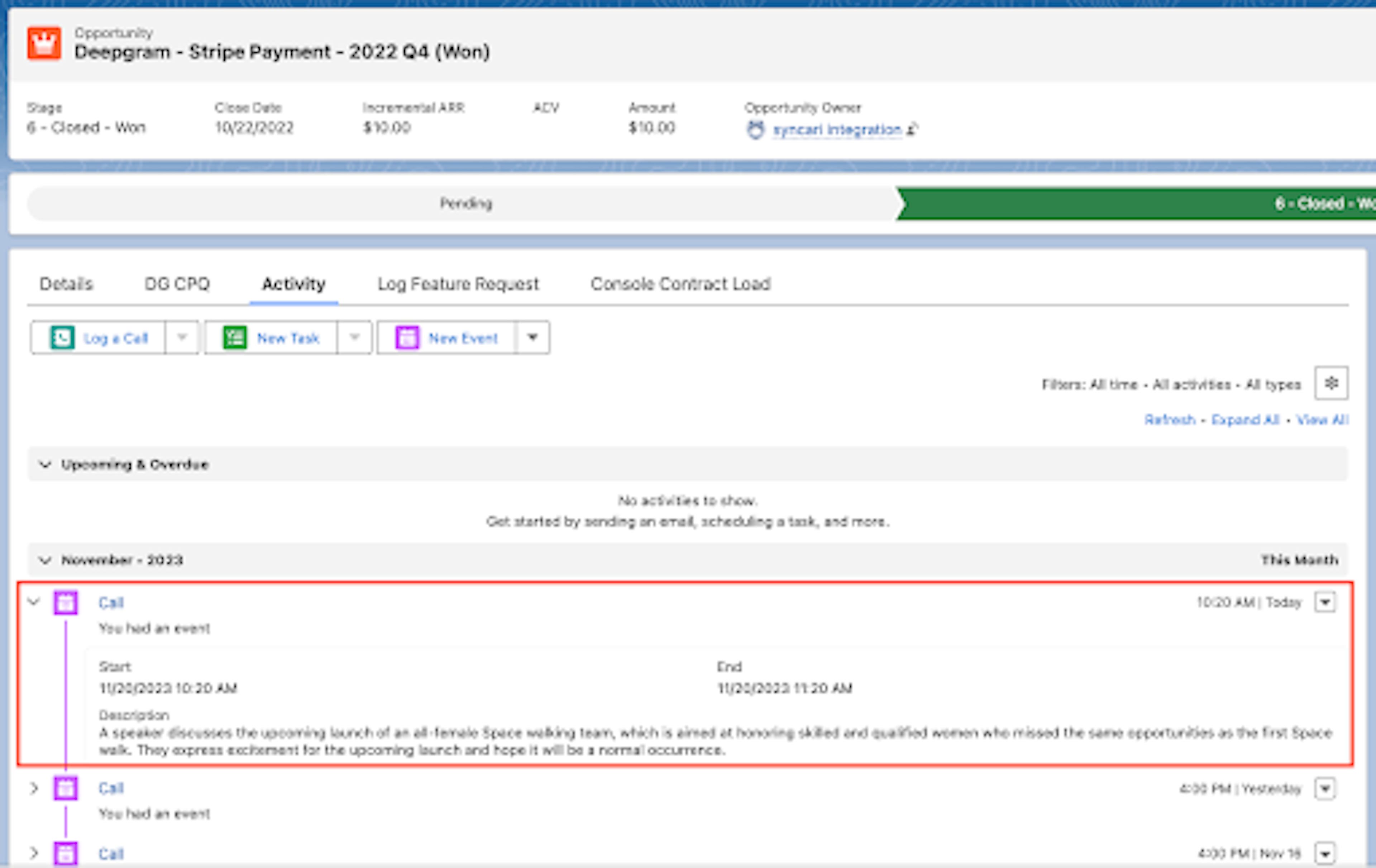
When you click on the call, you can view its full details, including the opportunity it is related to. Note that you can enrich this record when you create it by making use of any other Salesforce-provided fields, such as the location, other relationships, or reminders. You can also create custom fields for any additional metadata you want to log.
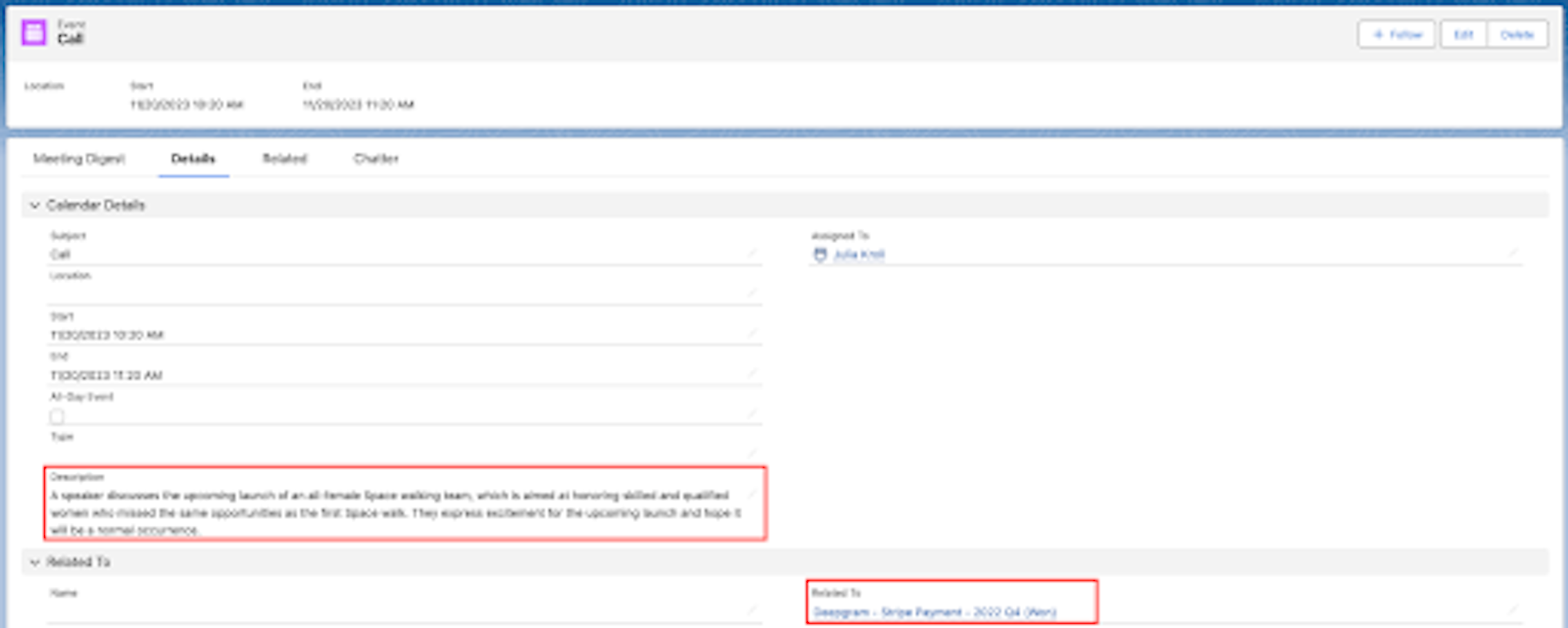
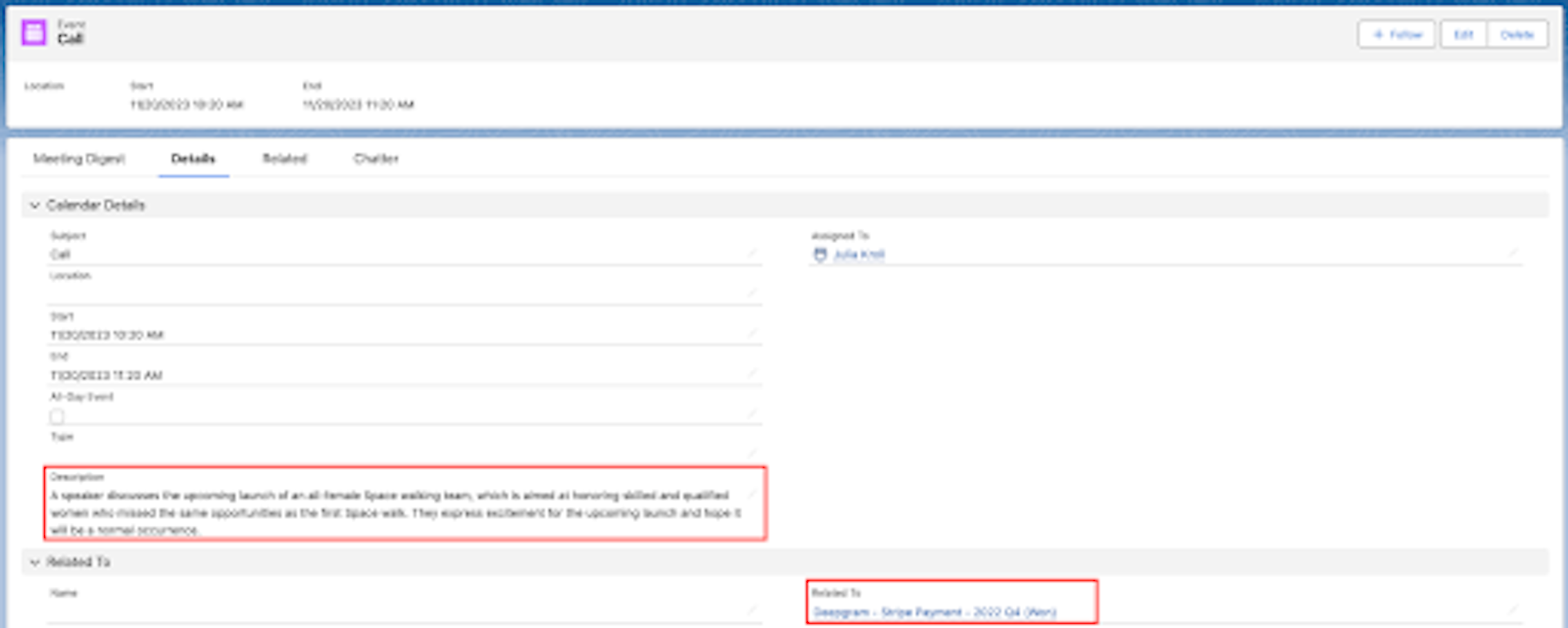
Conclusion
This blog post demonstrated how you can use the Deepgram Python SDK and the simple-salesforce Python package for the Salesforce Apex API in order to summarize a sales call and log its summary to a Salesforce opportunity and account. This code also offers ample room for additional extension and customization depending on how your company is using Salesforce.
If you have any feedback on this example, please be sure to drop us a note on our GitHub discussion forum, Discord, or Twitter.
Sign up for Deepgram
Sign up for a Deepgram account and get $200 in Free Credit (up to 45,000 minutes), absolutely free. No credit card needed!
Learn more about Deepgram
We encourage you to explore Deepgram by checking out the following resources:
Appendix: Complete code
Unlock language AI at scale with an API call.
Get conversational intelligence with transcription and understanding on the world's best speech AI platform.